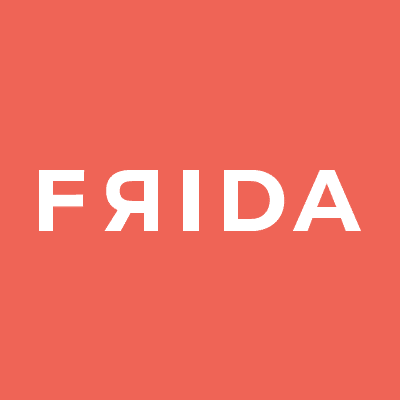
Patching an iOS application with Frida involves injecting custom code into the application’s runtime to modify its behavior. Tools like Hopper, Ghidra, and others can typically be used to modify an application’s opcode and patch it but this is another topic.
Demo
In the upcoming demonstration, we will be patching an iOS application that verifies if the device is jailbroken. This will involve utilizing NOP code on the bl instruction, which stands for Branch with Link.
As first thing we need to download the iOS application from here
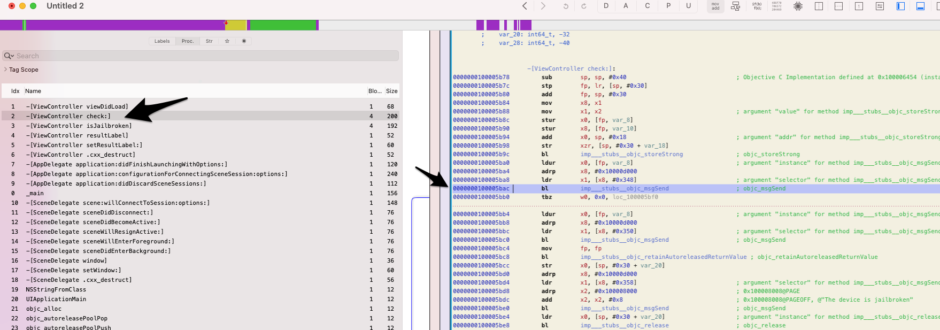
To achieve that we will use the following Frida script:
var targetModule=Process.enumerateModulesSync()[0].name
var addr=ptr(0x5bac);
var moduleBase=Module.getBaseAddress(targetModule);
var targetAddress=moduleBase.add(addr);
Memory.patchCode(targetAddress, 4, code => {
let cs = new Arm64Writer(code, { pc: code });
cs.putNop()
cs.flush();
});
Instead to use cs.putNop we could use the following instruction cs.putInstruction(0x1F2003D5) where 0x1F2003D5 is the equivalent of the Nop op code in Hex.
To convert arm instruction to hex and viceversa I usally use the following website: https://armconverter.com/?disasm&code=1F2003D5%0A
Other usefull links are:
- https://developer.arm.com/
- https://frida.re/docs/javascript-api/#armwriter